Adding RBAC with Terraform¶
In Observe, you can assign assign users to RBAC groups with Terraform, an open-source infrastructure as “code” development tool. To learn more about using Terraform, visit their Web site. For additional information on using Terraform with Observe, see Observe’s Terraform Provider Page.
Once you install Terraform, configure the provider, Observe, with the following attributes.
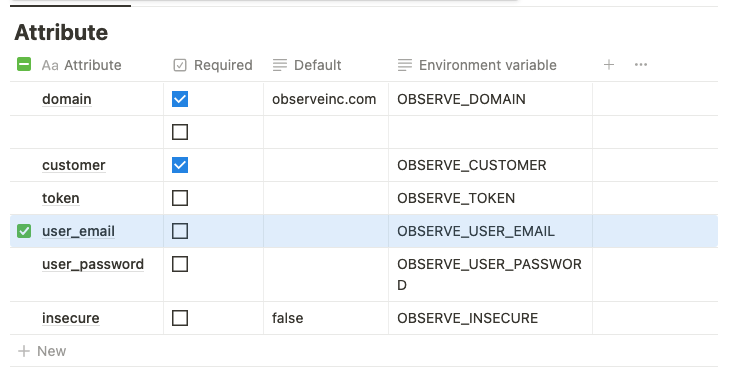
Figure 1 - Terraform attributes supported by Observe
Add the domain, observeinc.com, and your Observe customer ID in the customer field. Your customer ID precedes the domain name of your instance, for example, 123456789012.observeinc.com. 123456789012 is your customer ID.
Note
Some Observe instances may optionally use a name instead of Customer ID; if this is the case for your instance, contact your Observe Data Engineer to discuss implementation. A stem name will work as is, but a DNS redirect name may require client configuration.
You also need the following Terraform for your Observe setup:
Note
Your user must have Administrator
permissions in Observe to create or modify RBAC resources.
# provider.tf
provider "observe" {
customer = "123456789012"
domain = "observeinc.com"
user_email = "[email protected]"
user_password = "secret"
}
# main.tf
terraform {
required_providers {
observe = {
source = "terraform.observeinc.com/observeinc/observe"
version = "~> 0.11"
}
}
}
data "observe_workspace" "default" {
name = "Default"
}
As a general rule, users can view the contents of any dataset. However, you can create a more specific rule that only allows a user to view a particular Dataset.
When you set permissions using RBAC, grant only the permissions required to perform a task. You do this by defining the actions taken on specific resources under specific conditions, also known as least-privilege permissions. You might start with broader permissions while you explore the permissions required for your workload or use case. As your use case matures, you can work to reduce the permissions that you grant to work toward least privilege.
Consider a permission flow similar to the following flow:
Determine all rules that might apply to this access request.
Determine the most specific scope within these rules.
Determine the most permissive permission granted within that scope.
Using Terraform to Look Up Users and Groups¶
Look Up Users¶
To look up a user by email address or user ID, configure the following Terraform:
data "observe_user" "john_doe" {
email = "[email protected]"
}
data "observe_user" "user_id" {
id = "1234"
}
Replace the placeholder information with your user data.
Look Up RBAC Groups¶
To look up an RBAC Group, configure the following Terraform:
data "observe_rbac_group" "reader" {
name = "reader"
}
Managing RBAC Groups and Memberships¶
Manage your groups and memberships using the following Terraform:
Create an Engineering Group¶
resource "observe_rbac_group" "engineering" {
name = "Engineering"
}
Add a User to the Engineering Group¶
resource "observe_rbac_group_member" "johndoe_engineering" {
group = observe_rbac_group.engineering.oid
description = "add John to engineering group"
member {
user = data.observe_user.john_doe.oid
}
}
Add an Engineering Group to the Writer Group¶
resource "observe_rbac_group_member" "engineering_writers" {
group = data.observe_rbac_group.writer.oid
description = "add engineering group to writer group"
member {
group = observe_rbac_group.engineering.oid
}
}
Managing RBAC Statements Using Terraform¶
To control access, use the observe_rbac_statement
statement. Statements consist of the following components:
subject - statement applies to the user or group. For example, the statement applies to these subjects,
group
,user
, orall
.object - statement applies to one of the following:
id
workspace
type
all
You can optionally specify name
or owner
with type
.
Note
Use id
to target specific objects. Use workspace
to target all objects within those scopes.
role - specify one of the following roles:
Manager
- can manage the Observe roles.Editor
- can edit datasets and manipulate data.Viewer
- can view Datasets but cannot perform other actions.Lister
- can view the requested objects and details, including the configuration, about the object. Cannot query payload data from the requested object.
Restricting Access to Sensitive Data¶
# Create a sensitive readers group
resource "observe_rbac_group" "sensitive_readers" {
name = "Sensitive Readers"
}
Look Up a Sensitive Dataset¶
data "observe_dataset" "sensitive" {
workspace = data.observe_workspace.default.oid
name = "Sensitive Dataset"
}
Lock Down Access to a Sensitive Dataset¶
resource "observe_rbac_statement" "lock_sensitive_dataset" {
subject { all = true }
object { id = data.observe_dataset.sensitive.id }
role = "Lister"
}
Allowing Members of a sensitive readers
Group to View a Dataset¶
resource "observe_rbac_statement" "lock_dataset_readers" {
subject { group = observe_rbac_group.sensitive_readers.oid }
object { id = data.observe_dataset.sensitive.id }
role = "Viewer"
}
Allowing Specific Users to View a Dataset¶
resource "observe_rbac_statement" "john_sensitive_dataset" {
subject { user = data.observe_user.john_doe.oid }
object { id = data.observe_dataset.sensitive.id }
role = "Viewer"
}
Binding Users to the sensitive_readers
Group¶
resource "observe_rbac_group_member" "john_sensitive" {
group = data.observe_rbac_group.sensitive_readers.oid
member { user = data.observe_user.john_doe.oid }
}